Device registry
The device registry is a registry where Home Assistant keeps track of devices. A device is represented in Home Assistant via one or more entities. For example, a battery-powered temperature and humidity sensor might expose entities for temperature, humidity and battery level.
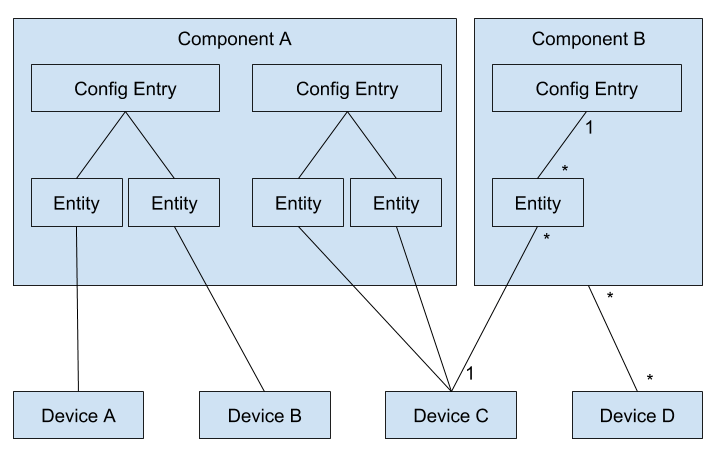
What is a device?
A device in Home Assistant represents either a physical device that has its own control unit, or a service. The control unit itself does not have to be smart, but it should be in control of what happens. For example, an Ecobee thermostat with 4 room sensors equals 5 devices in Home Assistant, one for the thermostat including all sensors inside it, and one for each room sensor. Each device exists in a specific geographical area, and may have more than one input or output within that area.
If you connect a sensor to another device to read some of its data, it should still be represented as two different devices. The reason for this is that the sensor could be moved to read the data of another device.
A device that offers multiple endpoints, where parts of the device sense or output in different areas, should be split into separate devices and refer back to parent device with the via_device
attribute. This allows the separate endpoints to be assigned to different areas in the building.
Although not currently available, we could consider offering an option to users to merge devices.
Device properties
Attribute | Description |
---|---|
area_id | The Area which the device is placed in. |
config_entries | Config entries that are linked to this device. |
configuration_url | A URL on which the device or service can be configured, linking to paths inside the Home Assistant UI can be done by using homeassistant://<path> . |
connections | A set of tuples of (connection_type, connection identifier) . Connection types are defined in the device registry module. Each item in the set uniquely defines a device entry, meaning another device can't have the same connection. |
default_manufacturer | The manufacturer of the device, will be overridden if manufacturer is set. Useful for example for an integration showing all devices on the network. |
default_model | The model of the device, will be overridden if model is set. Useful for example for an integration showing all devices on the network. |
default_name | Default name of this device, will be overridden if name is set. Useful for example for an integration showing all devices on the network. |
entry_type | The type of entry. Possible values are None and DeviceEntryType enum members (only service ). |
hw_version | The hardware version of the device. |
id | Unique ID of device (generated by Home Assistant) |
identifiers | Set of (DOMAIN, identifier) tuples. Identifiers identify the device in the outside world. An example is a serial number. Each item in the set uniquely defines a device entry, meaning another device can't have the same identifier. |
name | Name of this device |
name_by_user | The user configured name of the device. |
manufacturer | The manufacturer of the device. |
model | The model name of the device. |
model_id | The model identifier of the device. |
serial_number | The serial number of the device. Unlike a serial number in the identifiers set, this does not need to be unique. |
suggested_area | The suggested name for the area where the device is located. |
sw_version | The firmware version of the device. |
via_device | Identifier of a device that routes messages between this device and Home Assistant. Examples of such devices are hubs, or parent devices of a sub-device. This is used to show device topology in Home Assistant. |
Defining devices
Automatic registration through an entity
Entity device info is only read if the entity is loaded via a config entry and the unique_id
property is defined.
Each entity is able to define a device via the device_info
property. This property is read when an entity is added to Home Assistant via a config entry. A device will be matched up with an existing device via supplied identifiers or connections, like serial numbers or MAC addresses. If identifiers and connections are provided, the device registry will first try to match by identifiers. Each identifier and each connection is matched individually (e.g. only one connection needs to match to be considered the same device).
# Inside a platform
class HueLight(LightEntity):
@property
def device_info(self) -> DeviceInfo:
"""Return the device info."""
return DeviceInfo(
identifiers={
# Serial numbers are unique identifiers within a specific domain
(hue.DOMAIN, self.unique_id)
},
name=self.name,
manufacturer=self.light.manufacturername,
model=self.light.productname,
model_id=self.light.modelid,
sw_version=self.light.swversion,
via_device=(hue.DOMAIN, self.api.bridgeid),
)
Besides device properties, device_info
can also include default_manufacturer
, default_model
, default_name
. These values will be added to the device registry if no other value is defined just yet. This can be used by integrations that know some information but not very specific. For example, a router that identifies devices based on MAC addresses.
Manual registration
Components are also able to register devices in the case that there are no entities representing them. An example is a hub that communicates with the lights.
# Inside a component
from homeassistant.helpers import device_registry as dr
device_registry = dr.async_get(hass)
device_registry.async_get_or_create(
config_entry_id=entry.entry_id,
connections={(dr.CONNECTION_NETWORK_MAC, config.mac)},
identifiers={(DOMAIN, config.bridgeid)},
manufacturer="Signify",
suggested_area="Kitchen",
name=config.name,
model=config.modelname,
model_id=config.modelid,
sw_version=config.swversion,
hw_version=config.hwversion,
)
Removing devices
Integrations can opt in to allow the user to delete a device from the UI. To do this, integrations should implement the function async_remove_config_entry_device
in their __init__.py
module.
async def async_remove_config_entry_device(
hass: HomeAssistant, config_entry: ConfigEntry, device_entry: DeviceEntry
) -> bool:
"""Remove a config entry from a device."""
When the user clicks the delete device button for the device and confirms it, async_remove_config_entry_device
will be awaited and if True
is returned, the config entry will be removed from the device. If it was the only config entry of the device, the device will be removed from the device registry.
In async_remove_config_entry_device
the integration should take the necessary steps to prepare for device removal and return True
if successful. The integration may optionally act on EVENT_DEVICE_REGISTRY_UPDATED
if that's more convenient than doing the cleanup in async_remove_config_entry_device
.
Categorizing to device info
Device info is categorized into Link, Primary and Secondary by finding the first device info type which has all the keys of the device info.
Category | Keys |
---|---|
Link | connections and identifiers |
Primary | configuration_url , connections , entry_type , hw_version , identifiers , manufacturer , model , name , suggested_area , sw_version , and via_device |
Secondary | connections , default_manufacturer , default_model , default_name , and via_device |
This categorization is used in sorting the configuration entries to define the main integration to be used by the frontend.
Mandatorily, the device info must match one of the categories.